There is one interesting difference between humans and computers. Most human beings with even a rudimentary grasp of mathematics can handle numbers quite happily. Some numbers may be harder to work with because of the size of them but, all in all, people know what to do with them.
However, while computers are very add up very quickly, sometimes they appear to do strange things numbers. A programmer must be aware that, to a computer, not all numbers are the same and that some programming languages can produce some quite surprising results. It is, therefore, worth looking at some of the pitfalls can can befall an application developer as they manipulate data in their programs.
An Unexpected Output
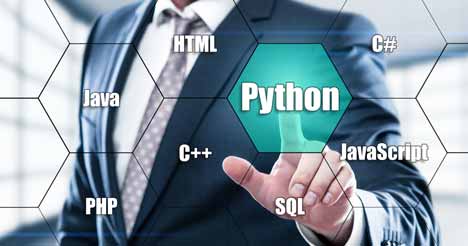
A Java programmer, for example, may decide that the int (or integer) number type may be suitable for creating id numbers, for example:
int myId;
myId = 2147483646;
myId++; System.out.println (myId);
The new id is obviously 2147483647 (as shown in Figure 1 at the bottom of this article). However, something strange happens when then next id is generated:
int myId;
myId = 2147483647;
myId++; System.out.println (myId);
This time the answer is not 2147483648 as any human being would expect. It is -2147483648 (as shown in Figure 2). This is not some strange new kind of mathematics. It’s simply that the computer does not see the number in the same way that a human does. It sees it as an allocation of memory.
Numbers and Memory
Most programming languages will support the number types:
- short (16 bits) – signed integer (-32768 to 32767)
- int (32 bits) – signed integer (-2147483648 to 214783647)
- long (64 bits) – signed integer (-9223372036854775808 to 9223372036854775807)
- float (32 bits) – floating point (approximately -1038.53 to 1038.53 )
- double (64 bits) – floating point (approximately -10308.25 to 10308.25)
Some programming languages will support additional number definitions, for example Java supports the byte (8 bits) which is a signed integer from -128 to 217. And it’s worth noting that although some of the number ranges are very large, none of them are infinite.
A Better Solution
The programmer must, therefore, choose a number type appropriate for the data that they expect to use, for example:
long myId;
myId = 2147483646;
myId++;
myId++; System.out.println (myId);
This time the correct result will be displayed (a shown in figure 3).
Not All Programming Languages are the Same
Of course, not all programming languages are as concerned about the number type being used. VBScript (or Visual Basic Script) will assign and reassign the number type as required:
Dim myID: myID = 2147483646
myID = myID + 1
wscript.echo myID
myID = myID + 1 wscript.echo myID
Even though the programmer has not defined the the number type to be used, the correct number is calculated (as shown in figure 4). However, it must be remembered that:

- no number range is infinite
- an application that uses only doubles will handle number correctly but may use a lot of memory
- a program that uses only shorts will not use much memory but will only be able to handle small numbers
For the programmer, therefore, there is a trade off between the amount of the memory to be used and the size of the number that can be used. However, if they are aware of the limitations of the number types that they are using then they will be able to create an accurate and efficient number crunching application.